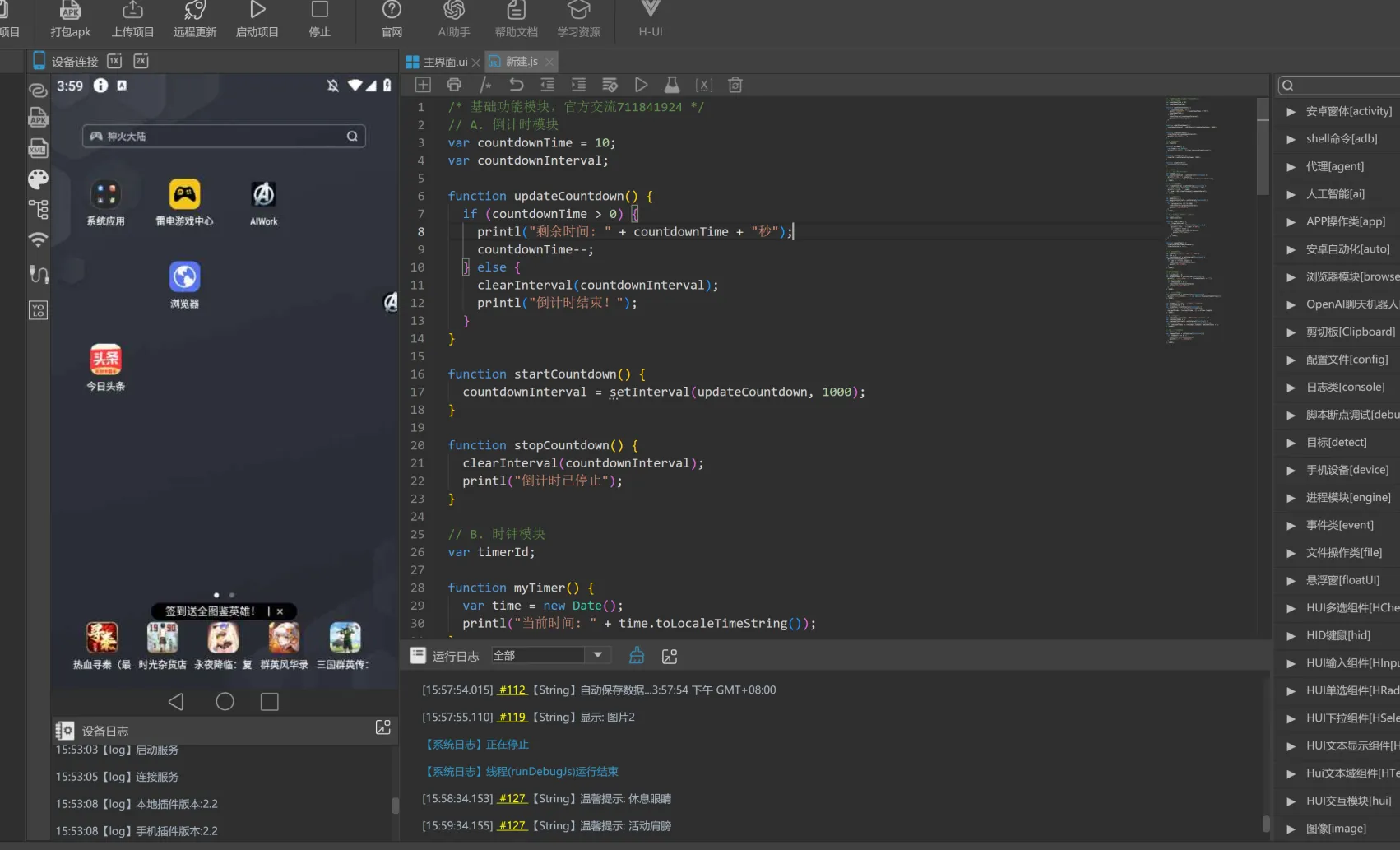
1. 倒计时功能
var countdownTime = 10;
var countdownInterval;
function updateCountdown() {
if (countdownTime > 0) {
console.log("剩余时间: " + countdownTime + "秒");
countdownTime--;
} else {
clearInterval(countdownInterval);
console.log("倒计时结束!");
}
}
用法
- 启动:
startCountdown()
- 停止:
stopCountdown()
应用场景
游戏倒计时、会议提醒、考试计时、烹饪计时等。
2. 实时时钟显示
let myVar;
function myTimer() {
const time = new Date().toLocaleTimeString();
console.log("当前时间:" + time);
}
function myStopFunction() {
clearInterval(myVar);
}
// 启动时钟
myVar = setInterval(myTimer, 1000);
// 停止时钟:myStopFunction()
扩展应用示例
1. 简单计数器
let counter = 0;
const intervalId = setInterval(() => {
console.log("计数:" + counter);
counter++;
if (counter > 10) {
clearInterval(intervalId);
console.log("计数停止");
}
}, 1000);
2. 随机数生成器
const intervalId = setInterval(() => {
const num = Math.floor(Math.random() * 100);
console.log("随机数:" + num);
if (num > 90) {
clearInterval(intervalId);
console.log("触发停止条件");
}
}, 1000);
3. 进度条模拟
let progress = 0;
const intervalId = setInterval(() => {
console.log("进度:" + progress + "%");
progress += 10;
if (progress >= 100) {
clearInterval(intervalId);
console.log("进度完成!");
}
}, 1000);
4. 倒计时简化版
let countdown = 10;
const intervalId = setInterval(() => {
console.log("倒计时:" + countdown + "秒");
countdown--;
if (countdown < 0) {
clearInterval(intervalId);
console.log("倒计时结束!");
}
}, 1000);
5. 循环输出数组
const arr = ["苹果", "香蕉", "橙子", "葡萄"];
let index = 0;
const intervalId = setInterval(() => {
console.log("当前水果:" + arr[index]);
index++;
if (index >= arr.length) {
clearInterval(intervalId);
console.log("所有水果已输出");
}
}, 1000);
结构说明
- 分级标题:使用
基础功能示例
和扩展应用示例
分类,便于快速定位场景 - 代码块统一:所有示例采用标准JavaScript语法,保留核心逻辑
- 关键注释:在倒计时示例中保留变量单位说明(
// 单位:秒
) - 场景提示:在基础功能中明确列出应用场景,增强实用性
可根据实际需求调整时间间隔、判断条件或输出内容。
/* 基础功能模块,官方交流711841924 */
// A. 倒计时模块
var countdownTime = 10;
var countdownInterval;
function updateCountdown() {
if (countdownTime > 0) {
printl("剩余时间: " + countdownTime + "秒");
countdownTime--;
} else {
clearInterval(countdownInterval);
printl("倒计时结束!");
}
}
function startCountdown() {
countdownInterval = setInterval(updateCountdown, 1000);
}
function stopCountdown() {
clearInterval(countdownInterval);
printl("倒计时已停止");
}
// B. 时钟模块
var timerId;
function myTimer() {
var time = new Date();
printl("当前时间: " + time.toLocaleTimeString());
}
function startClock() {
timerId = setInterval(myTimer, 1000);
}
function stopClock() {
clearInterval(timerId);
}
/* 实用案例 */
// 1. 简单计数器(1-10)
var counter = 1;
var counterInterval = setInterval(function() {
printl("计数: " + counter);
if (counter++ >= 10) clearInterval(counterInterval);
}, 1000);
// 2. 随机数生成器
var randomInterval = setInterval(function() {
var num = Math.floor(Math.random() * 100);
printl("随机数: " + num);
if (num > 90) clearInterval(randomInterval);
}, 1000);
// 3. 进度条模拟
var progress = 0;
var progressInterval = setInterval(function() {
printl("进度: " + progress + "%");
if ((progress += 10) >= 100) {
clearInterval(progressInterval);
printl("进度完成!");
}
}, 1000);
// 4. 增强版倒计时(带暂停恢复)
var timer = 10;
var timerInterval;
function startTimer() {
if (!timerInterval) {
timerInterval = setInterval(function() {
printl("剩余: " + timer + "秒");
if (timer-- <= 0) {
clearInterval(timerInterval);
printl("时间到!");
}
}, 1000);
}
}
function pauseTimer() {
clearInterval(timerInterval);
timerInterval = null;
}
// 5. 数组遍历输出
var fruits = ["苹果", "香蕉", "橙子"];
var idx = 0;
var fruitInterval = setInterval(function() {
printl(fruits[idx++]);
if (idx >= fruits.length) {
clearInterval(fruitInterval);
printl("输出完成");
}
}, 1000);
/* 新增ES5案例 */
// 6. 网络状态检测
var checkCount = 0;
var networkCheck = setInterval(function() {
printl("检测网络状态...(" + (++checkCount) + ")");
// 模拟网络恢复
if (checkCount > 3) {
clearInterval(networkCheck);
printl("网络已连接!");
}
}, 2000);
// 7. 自动保存功能
var saveInterval = setInterval(function() {
printl("自动保存数据..." + new Date().toLocaleTimeString());
}, 5000);
// 8. 轮播图控制
var slides = ["图片1", "图片2", "图片3"];
var currentSlide = 0;
var slideShow = setInterval(function() {
printl("显示: " + slides[currentSlide]);
currentSlide = (currentSlide + 1) % slides.length;
}, 3000);
// 9. 定时提醒器
var reminders = ["喝水", "休息眼睛", "活动肩膀"];
var reminderIndex = 0;
var reminderInterval = setInterval(function() {
printl("温馨提示: " + reminders[reminderIndex++]);
if (reminderIndex >= reminders.length) reminderIndex = 0;
}, 60000);
// 10. 任务超时监控
var timeout = 5;
var timeoutCheck = setInterval(function() {
if (timeout-- <= 0) {
clearInterval(timeoutCheck);
printl("任务执行超时!");
}
}, 1000);
应用场景扩展:
- 表单自动保存(案例7)
- 轮播图自动切换(案例8)
- 健康提醒系统(案例9)
- 接口超时监控(案例10)
- 网络重连机制(案例6)
- 演示文稿自动播放(案例8修改)
- 操作步骤引导(案例5扩展)
- 游戏技能冷却时间(案例4修改)
- 考试时间提醒(案例4+9结合)
- 数据同步监控(案例10扩展)
每个示例都遵循以下ES5特性:
- 使用
var
声明变量 - 使用
function
关键字定义函数 - 使用传统函数表达式代替箭头函数
- 字符串拼接使用
+
运算符 - 使用传统数组遍历方式
- 基于原型的对象操作
可以通过调用对应函数(如 startTimer()/pauseTimer())或直接运行定时器来使用这些功能。所有定时器都支持通过 clearInterval() 提前终止。